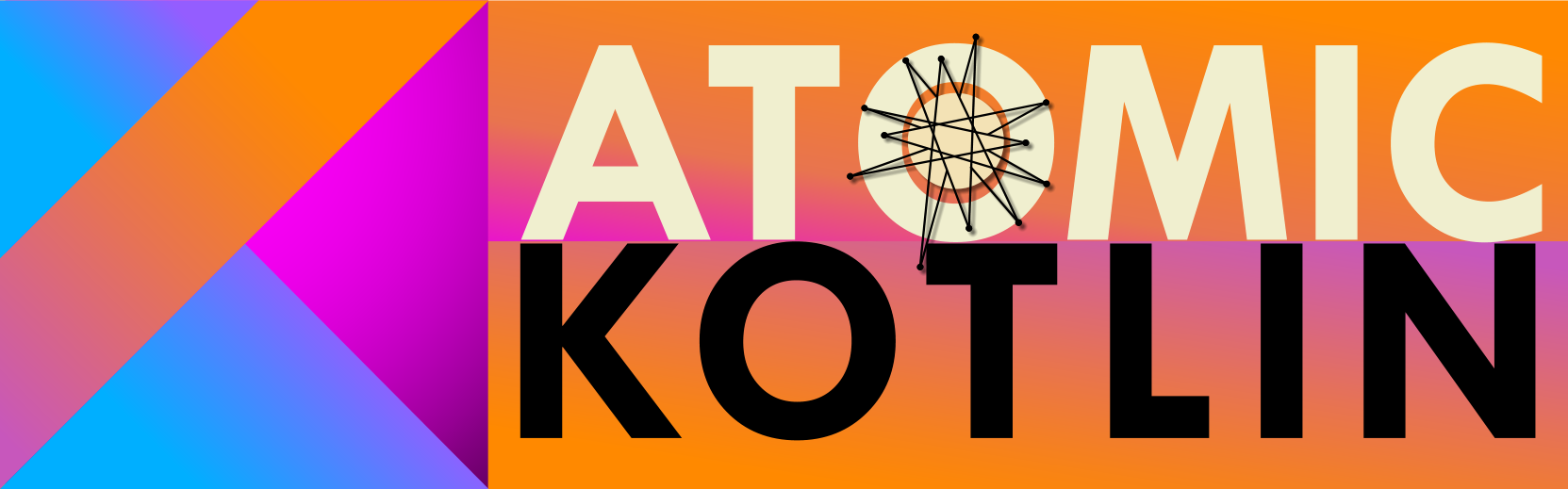
Table of Contents
This shows the table of contents for the completed book.
The bold & italicized atoms in the table of contents below are those included in the Leanpub Free Sample. The Stepik Free Sample includes beginning portions of all non-bold & italicized atoms.
Section I: Programming Basics
- Introduction
- Why Kotlin?
- Hello, World!
var
&val
- Data Types
- Functions
if
Expressions- String Templates
- Number Types
- Booleans
- Repetition with
while
- Looping & Ranges
- The
in
Keyword - Expressions & Statements
Summary 1
Section II: Introduction to Objects
Objects Everywhere
Creating Classes
Properties
Constructors
Constraining Visibility
Packages
Testing
Exceptions
Lists
Variable Argument Lists
Sets
Maps
Property Accessors
Summary 2
Section III: Usability
Extension Functions
Named & Default Arguments
Overloading
when
ExpressionsEnumerations
Data Classes
Destructuring Declarations
Nullable Types
Safe Calls & the Elvis Operator
Non-Null Assertions
Extensions for Nullable Types
Introduction to Generics
Extension Properties
break
&continue
Section IV: Functional Programming
Lambdas
The Importance of Lambdas
Operations on Collections
Member References
Higher-Order Functions
Manipulating Lists
Building Maps
Sequences
Local Functions
Folding Lists
Recursion
Section V: Object-Oriented Programming
Interfaces
Complex Constructors
Secondary Constructors
Inheritance
Base Class Initialization
Abstract Classes
Upcasting
Polymorphism
Composition
Inheritance & Extensions
Class Delegation
Downcasting
Sealed Classes
Type Checking
Nested Classes
Objects
Inner Classes
Companion Objects
Section VI: Preventing Failure
Exception Handling
Check Instructions
The
Nothing
TypeResource Cleanup
Logging
Unit Testing
Section VII: Power Tools
Extension Lambdas
Scope Functions
Creating Generics
Operator Overloading
Using Operators
Property Delegation
Property Delegation Tools
Lazy Initialization
Late Initialization
Appendices
Appendix A: AtomicTest
Appendix B: Java Interoperability